FAQs
One of our most frequently asked remote monitoring questions is “can I get an email alert when something happens?”
This is something you can do using any Brainboxes ED device and the standard lights or sensors on your production line. You will need some familiarity with C# programming to follow the annotated example below. If you need help with creating a more complex application, Brainboxes work with some excellent partners who can assist – talk to our Sales team.
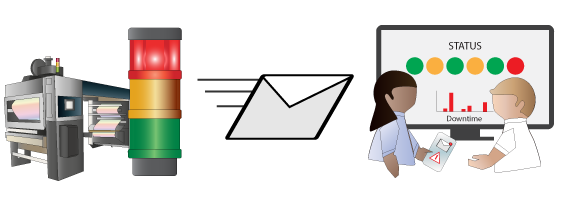
This FAQ will show you how to send an email when something happens on your production line. We can use the state change of digital inputs or outputs, so when something is pressed or triggered, such as an alarm, stop button or light. We can also count a number of events, that for example could represent finished goods, and then send an email when a target is reached.
Here are some potential scenarios:
- Connect an input to a button, so that production line staff can send a message when maintenance is required
- Connect to the alarm light on a machine, an email will be sent when the alarm is asserted
- Connect to the proximity sensor of a machine, send email when number of units produced hits a target
- Connect to an analogue sensor monitoring fluid level, send email when level is too low and more is required
The email is sent over SMTP, using your email server. You will need a Brainboxes Remote IO device (product codes ED-xxx), and a Windows/Linux or Mac machine which is on the same network as the brainboxes ED device. You will need to modify the example C# code with the particulars of your application.
Send Email on Changing of an IO Line on an ED Device
The following code is written in C# which can be run on Windows/Linux or Mac:
using System;
using Brainboxes.IO; // install brainboxes.io api using nuget
using MailKit.Net.Smtp; // install mailkit using nuget
using MailKit;
using MimeKit;
namespace edEmailproject
{
class Program
{
static void Main(string[] args)
{
// update this value to the IP address of your ED- device
string ipAddress = "192.168.0.85";
EDDevice ed = EDDevice.Create(ipAddress);
Console.WriteLine($"Connected to {ed} at address {ipAddress}");
// send email if an input goes low, e.g. if a machine alarm is activated
Console.WriteLine("Monitoring Digital Input Line 0 for when it goes from high to low, send email when it happens");
ed.Inputs[0].IOLineFallingEdge += IOChangeSendEmail;
// alternatively wait for the count to reach a target value
Console.WriteLine("Monitoring Digital Input Line 1 for when the line goes low 100 times");
ed.Inputs[0].IOLineCount += (line, device, changeType) =>
{
if(line.Count > 100) {
SendEmail("Target of 100 Reached", "The production target of 100 units has been reached");
}
};
// if you are using an analogue input device (e.g. ED-549)
// connect it to an height/weight sensor
// send an email when the target hits the low level
AIOLineChangedEventHandler ioGoneBelowMinimumEventHandler = IOGoneBelowMinimum;
// the target value is 0.5, this is the analog input value expressed in volts or amps depending on the sensor type
ed.Inputs[2].SubscribeToTargetEvent(ref ioGoneBelowMinimumEventHandler, 0.5);
Console.WriteLine("Press any key to exit");
Console.ReadKey();
Console.WriteLine("Key Pressed, disconnecting and exiting!");
ed.Disconnect();
}
//when the level goes above a target level, send an email
private static void IOGoneBelowMinimum(IOLine line, EDDevice device, double value, AIOChangeTypes changeType)
{
//previous sampled value was above the target value and current sampled value is now below the target value
if(changeType == AIOChangeTypes.Below)
{
Console.WriteLine($"Level is below target value: {value}");
SendEmail("Liquid Below Minimum", "Please Top up amount of liquid in store 3");
}
}
private static void IOChangeSendEmail(IOLine line, EDDevice device, IOChangeTypes changeType)
{
Console.WriteLine($"Line {line} changed from high to low sending email");
SendEmail("The Alarm on Machine 2 was activated", "Please send a maintenance engineer to review machine 2 urgently");
}
private static void SendEmail(string subject, string message) {
var message = new MimeMessage ();
message.From.Add (new MailboxAddress ("Joey Tribbiani", "[email protected]"));
message.To.Add (new MailboxAddress ("Mrs. Chanandler Bong", "[email protected]"));
message.Subject = subject;
message.Body = new TextPart ("plain") {
Text = message
};
using (var client = new SmtpClient ()) {
// For demo-purposes, accept all SSL certificates (in case the server supports STARTTLS)
client.ServerCertificateValidationCallback = (s,c,h,e) => true;
client.Connect("smtp.myserver.com", 587, false);
// Note: only needed if the SMTP server requires authentication
client.Authenticate("joey", "password");
client.Send(message);
client.Disconnect(true);
}
Console.WriteLine("Email Sent");
}
}
}
For more information on using Brainboxes .NET API to create programs talking to brainboxes products see this FAQ.
Use NuGet in VS Code to install MailKit: https://www.nuget.org/packages/MailKit/ MailKit is the dot net core library used to connect to mail servers and send email.
The MailKit library works with lots of different email servers, for example to work with Gmail simple swap out the `using(var client = new SmtpClient()) { ` block in the example above with the following (note Google Specify that you use OAuth as the connection method):
using (var client = new SmtpClient ()) {
client.Connect ("smtp.gmail.com", 587);
// use the OAuth2.0 access token obtained by following: https://developers.google.com/identity/protocols/OAuth2
var oauth2 = new SaslMechanismOAuth2 ("[email protected]", credential.Token.AccessToken);
client.Authenticate (oauth2);
client.Send (message);
client.Disconnect (true);
}
Related FAQs
- Example code in C# for communicating using TCP with an ES device
- How do I count boxes?
- How do I create a windows forms application for Brainboxes Remote IO in C#?
- How do I use C# to communicate with my Analog IO Module?
- How do I use C# to communicate with my Remote IO Module?
- How do I use C# to control my Ethernet to Serial device?